New to vibe coding? Check out our intro blog: Secure Vibe Coding Made Simple.
Nothing is more exciting than realizing your vision. Nothing is worse than realizing you had a major security flaw.
The good news? While security is crucial, it doesn't have to be an obstacle, especially when you're building on Replit. We integrate many security features directly into the platform, and our AI tools, Agent and Assistant, can handle much of the heavy lifting, letting you focus on building.
This post is your practical guide to navigating security essentials. We'll provide a checklist and 16 practical tips for how you can vibe code more secure apps (and how to do so simply).
Your vibe code security checklist
Before diving into details, here's the complete security checklist you can use for your projects. Follow these 16 key practices to significantly reduce security risks in your applications: you can find the checklist in markdown here.
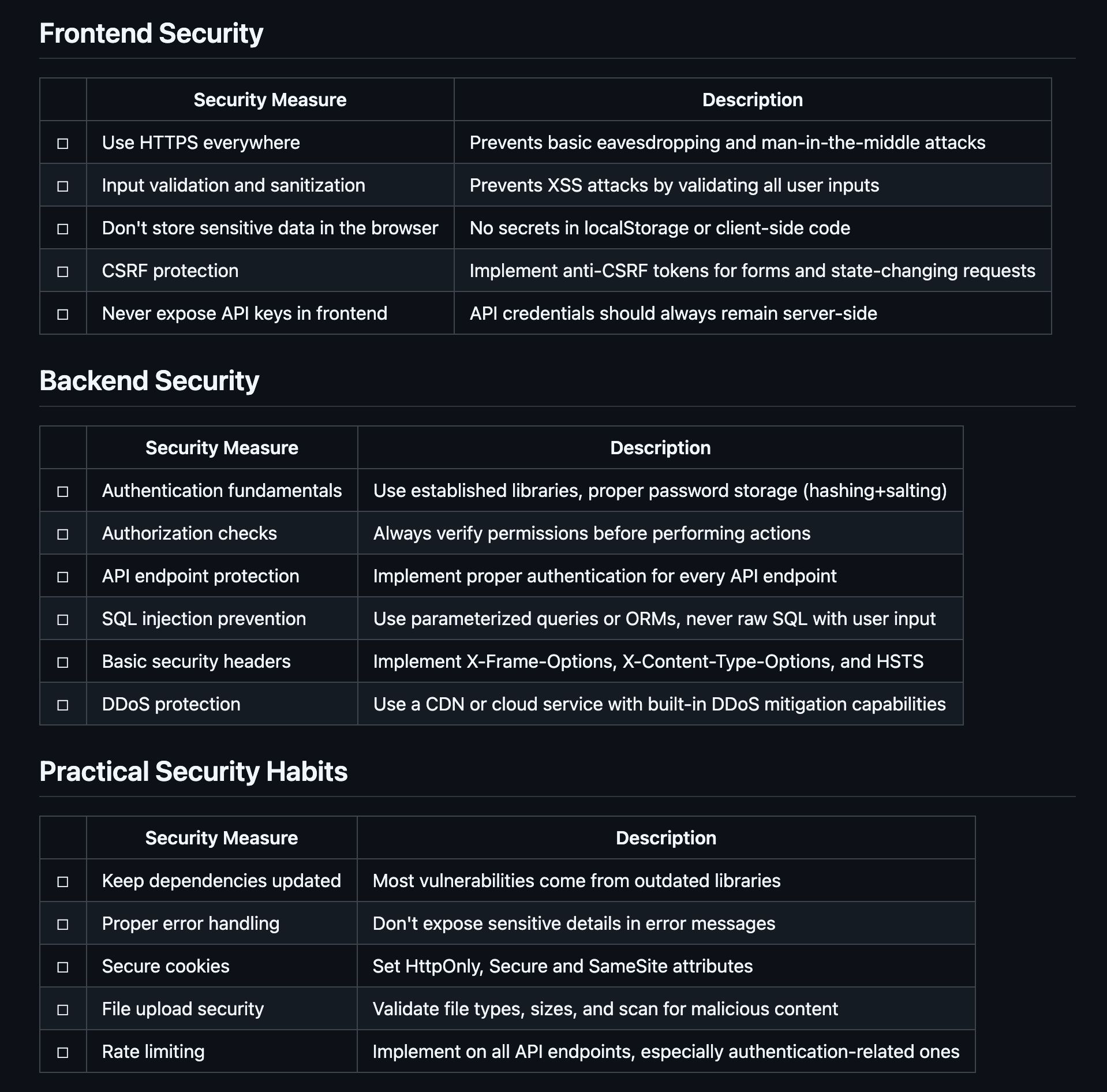
Now, let's walk through each of these points in detail, explaining why they matter and how Replit helps you address them.
Front-end fundamentals
The "front-end" of an app is the part that the user engages with. For example, in the app below the "frontend" is the solar system app being displayed.
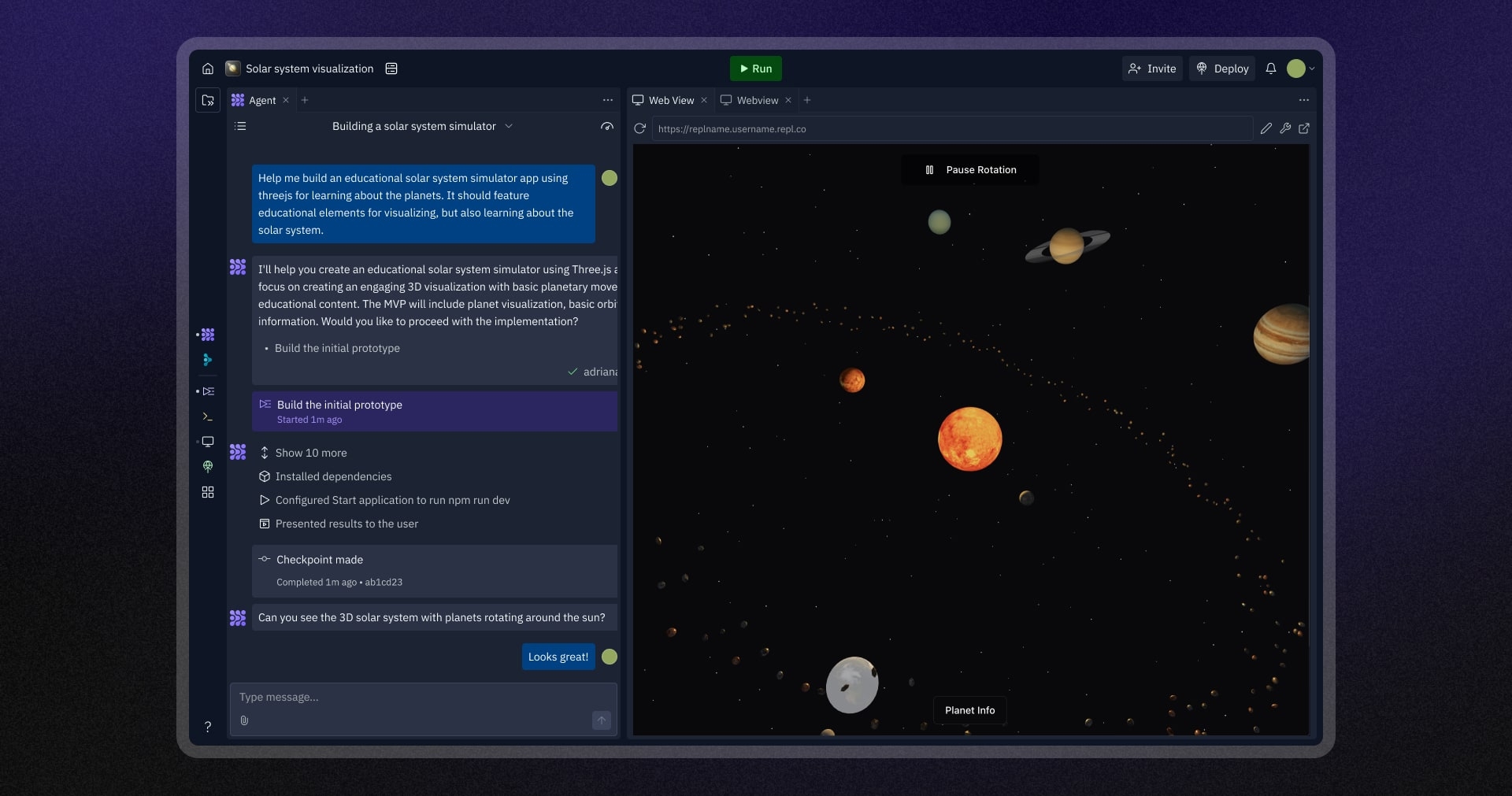
Data on the frontend is inherently insecure. Everything that gets passed around in frontend code is accessible, even if not explicitly visible.
That's because it's easy to inspect a web page for all of the information that's being transmitted. As such, we need to secure the frontends for our apps and avoid exposing sensitive information.
Here are some tips for secure frontend apps.
Always use HTTPS
Think of HTTPS as a secure, encrypted tunnel for data traveling between the user's browser and your app—preventing eavesdropping. It's a standard best practice.
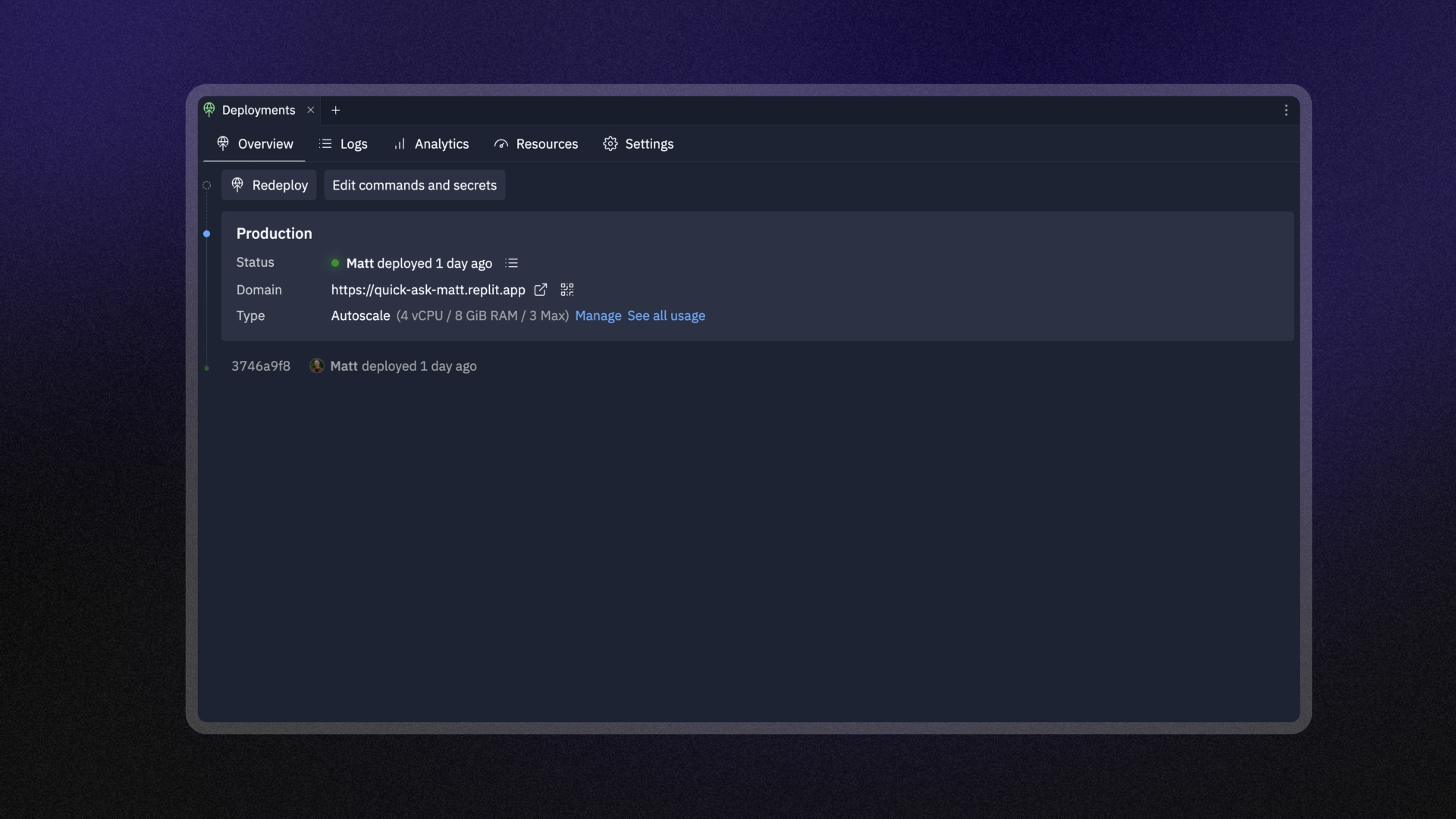
Replit Advantage: Apps deployed on Replit use HTTPS by default. We manage the certificates and setup, so there's no extra configuration needed from you.
Validate and sanitize user input
Never trust data coming directly from a user without checking it. Malicious actors might try to inject harmful code (like in Cross-Site Scripting (XSS) attacks) through forms or other inputs. Always validate (is the format correct?) and sanitize (remove potentially harmful elements).
How Replit Helps: While good front-end checks are important, Replit's back-end often uses ORMs (Object-Relational Mappers) which automatically help sanitize data before it reaches your database.
AI Assist: Give Assistant or Agent a nudge: "Validate and sanitize inputs in my user profile form to protect against XSS attacks." Remember to review the code it generates.
Keep secrets out of the browser
Your API keys, tokens, database passwords—this information is sensitive.
It should never live in your front-end JavaScript code or be stored in browser storage (like localStorage). Anyone can potentially inspect browser code and network requests.
Replit Advantage: Unlike other AI coding apps that outsource backends to 3rd parties, Replit Agent architects apps with a separate front-end and back-end. Sensitive operations happen on the back-end, safely away from browser inspectors. Replit Secrets provide a secure, dedicated place to store these credentials without hardcoding them.
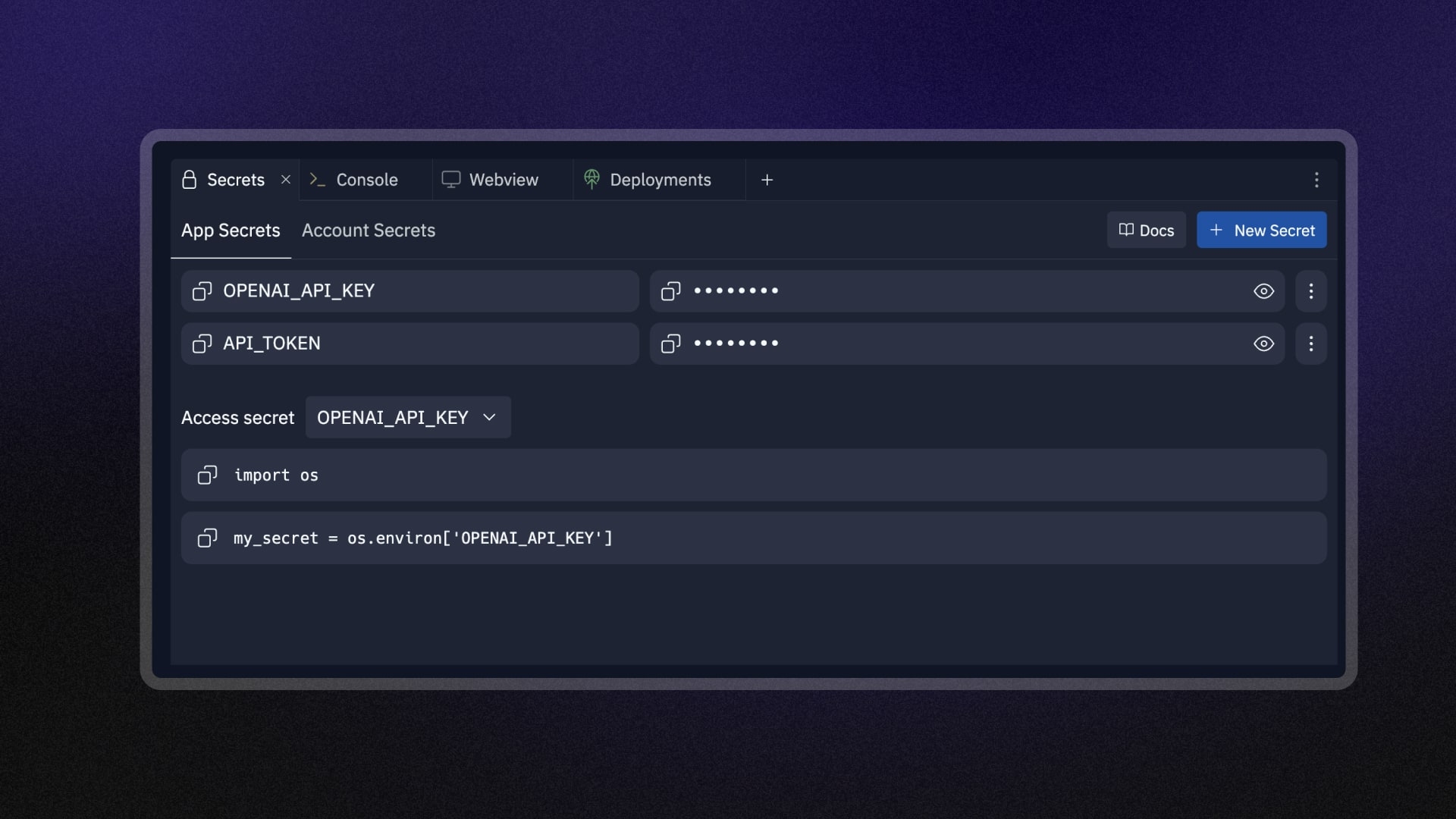
AI Assist: Feeling unsure? Ask Assistant: "Is my app storing any API keys or sensitive data in the front-end code?"
Protect against CSRF
Imagine a malicious website tricking a logged-in user's browser into performing actions on your site without their knowledge. That's Cross-Site Request Forgery (CSRF). The standard defense involves using unique, hidden tokens in your forms to verify the request's legitimacy.
AI Assist: This can seem technical, but AI can help simplify it. Try prompting: "Help me implement anti-CSRF tokens for the forms in my Flask app." As always, review the implementation.
Back-end best practices
The back-end is where your app's core logic runs, databases are accessed, and sensitive operations occur. Since the backend is hidden from the user, it's more secure than the frontend.

Replit Agent is the only coding agent that builds fullstack (frontend + backend) apps by default. That makes them more secure—sensitive data is processed in a secure, hidden environment.
Authentication: Verifying identity
Handling logins securely is critical. Avoid building your own login system from scratch unless absolutely necessary—it's complex to get right. Use established libraries, and never store passwords as plain text.
Replit Advantage: We've got you covered with Replit Auth: it lets users log in securely using their existing Replit account, simplifying the process while we manage the secure storage and verification (like "log in with Google" buttons, but on Replit).
AI Assist: Building with Agent? Just ask it to: "Use Replit Auth for user authentication." If you need to store other sensitive user info directly, always hash and salt it.
Prompt: "Ensure any sensitive user profile data stored in the database is securely hashed and salted." Remember: Handling user data, especially Personally Identifiable Information (PII), requires care—always protect user trust.
Authorization: Controlling access
Authentication confirms identity; authorization confirms permissions (what someone is allowed to do). Ensure users can only access the data and perform the actions appropriate for their role.
Replit Advantage: Apps built by Agent using Replit Auth usually include basic authorization checks.
Attention Needed: This requires diligence as your app grows. Always verify permissions before performing any action or showing sensitive data. Ask yourself: "Does this function check if the logged-in user actually owns this resource before modifying it?"
Protect your APIs
If your back-end provides APIs—even just for your own front-end—protect them. Unauthenticated APIs are open invitations. Use API keys, authentication tokens, or configure CORS (Cross-Origin Resource Sharing) correctly to ensure only authorized clients can access them.
AI Assist: This depends heavily on your app's structure. Ask Assistant: "How can I properly authenticate the API endpoints in my Express.js app to ensure only logged-in users can access them?"
Prevent SQL injection
A classic attack where malicious SQL commands are slipped into user inputs, potentially allowing attackers to steal or corrupt your database. ORMs (Object-Relational Mappers) automatically sanitize data and validate calls to your database, rendering SQL injection a thing of the past.
Replit Advantage: Agent always uses an ORM when building apps with databases. ORMs act as a safe layer, translating your code into SQL and using parameterized queries that prevent raw, malicious input from reaching the database engine. Learn more about Replit's database options here.

Implement standard security headers
Think of these as instructions you send to the user's browser via HTTP headers. Headers like X-Frame-Options, X-Content-Type-Options, and the powerful Content-Security-Policy help mitigate risks like clickjacking and certain types of XSS attacks.
AI Assist: Assistant can help set these up. Try: "Implement recommended security headers like X-Frame-Options and a basic Content-Security-Policy in my app's HTML response." You can test your site's headers with tools like Security Headers, but implement policies carefully—overly strict ones can sometimes affect legitimate app features.
DDoS protection
Distributed Denial of Service (DDoS) attacks attempt to overwhelm your app with traffic, making it unavailable for legitimate users.
Replit Advantage: Apps deployed on Replit are automatically protected against common DDoS attacks by Google Cloud Armor, part of our underlying infrastructure.
Practical tips
Security isn't just about code; it's also about process and attention to detail. The following practices help maintain security over time.
Keep dependencies updated
Software libraries get updated frequently, often to patch security vulnerabilities. Outdated dependencies are a significant source of risk.
AI Assist: Ask Assistant: "Are any of my Python packages in requirements.txt outdated or known to have security vulnerabilities? Can you help me update them safely?"
Handle errors gracefully
When things go wrong, make sure your error messages don't reveal sensitive internal details (like database credentials or full file paths) to the end-user or in browser console logs. Log detailed errors securely on the back-end for debugging.
AI Assist: Do a sanity check: "Review my error handling code. Am I potentially leaking sensitive system information in error messages shown to the user or logged in the console?"
Use secure cookies
If you use cookies for sessions, set the HttpOnly, Secure, and SameSite attributes correctly. This helps prevent common cookie-based attacks.
Replit Advantage: Using Replit Auth simplifies session management and helps handle this securely.
AI Assist: If you're managing cookies yourself: "Help me configure secure cookie settings (HttpOnly, Secure, SameSite=Lax) for my application's session cookies."
Secure file uploads
Allowing users to upload files requires caution. Validate file types (don't let someone upload PDFs if you only expect images), limit sizes, and ideally, scan files if possible. Store them securely, for example, in Replit Object Storage.
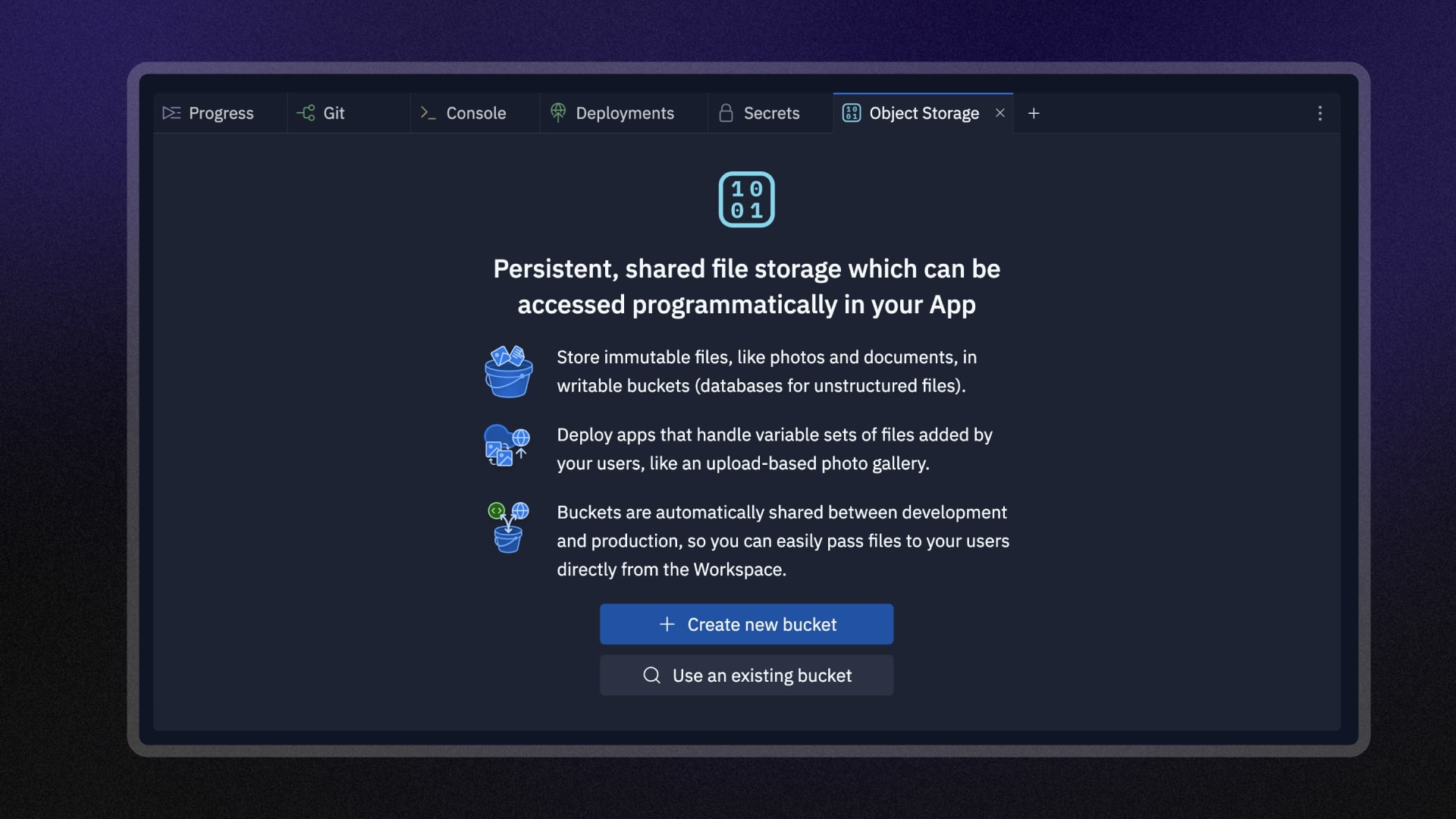
Replit Advantage: Files in Object Storage aren't directly executable on the server and have restricted access controls by default, enhancing security.
AI Assist: Prompt: "How can I securely handle image uploads in my app, ensuring only valid image types (JPEG, PNG) under 5MB are accepted?"
Apply rate limiting
Protect endpoints prone to abuse (like login forms) from brute-force attacks by limiting how many times a single user or IP address can make requests within a given time period.
AI Assist: Ask Assistant: "Help me implement basic rate limiting on my user login API endpoint to prevent brute-force attacks."
Understand your app's flow
An often overlooked aspect of security is simply understanding how data moves through your application. When you have a clear mental model of your app's architecture, you can better identify potential vulnerability points.
Replit Advantage: Agent creates well-structured applications with clear data flows. Ask Assistant to help explain any complex interactions you're not sure about: "Can you explain the data flow when a user submits this form in my application?"
Building secure is building smart
This might seem like a lot, but remember:
- Replit simplifies security. Features like default HTTPS, DDoS protection, secure Secret management, and Agent's use of ORMs handle significant parts automatically.
- AI is your partner. Use Assistant to check code, implement defenses, and learn about security concepts as you build.
- Focus on the fundamentals. Validating input, ensuring proper authentication/authorization, and keeping secrets safe are foundational.
Security isn't about achieving perfection overnight; it's about being proactive and informed. By understanding these core principles and leveraging the tools Replit provides, you can significantly reduce risk and build amazing applications with more confidence.
So go ahead, keep this checklist handy for your next project, and focus on the joy of creation—knowing you've covered the security essentials.